Use of variables
Variables make it possible to store values and to use these values as operands for mathematical and logical expressions. Variables can be used for calculating a variable position, an offset, or as a value for address letters, for example, as follows in any G-code dialect:
WaitTime = Real1 + 500
G1 X100 + Offset_X Y100 + Offset_Y F=Fast
G1XPosition_X YPosition_Y
G4 Time=WaitTime
TargetReached = TRUE
When assigning values or variables to other variables in the G-code, it is mandatory to specify an equal sign as follows:
Real1 = 100, Real1 = Real2, Bool1 = True, Bool1 = Bool2,
Pose1[3] = 10, Pose[3] = Real1.
For address letters, specifying the equal sign as an assignment is optional:
X100
is equivalent toX=100
XReal1
is equivalent toX=Real1
XReal1 + 100
is equivalent toX=Real1+100
In G-code, the same data types (Bool, Real and Pose) can be used as in SRL programming. In SRL programming, variables are defined and used by their index. In G-code, defining and using is done by their names. In order to convert G-code to SRL commands and vice versa, it is necessary to define which G-code variable uses which index and type of SRL variable. The user must make this assignment of G-code to SRL variable in the Configuration variables menu before importing or exporting. In this mapping table, the name of the G-code variable is mapped to the configured index of the SRL variable during import. Conversely, during the export of G-code, the variable index used in the SRL program is assigned to the G-code name. Variables cannot be imported or exported without a defined assignment in the assignment table. Unknown strings or undefined variables lead to syntax errors. G-code variables are globally valid for any program by means of this list, and can be used in any G-code program (as well as in other SRL programs and in CallFunctions by the index of the SRL variables).
With an existing G-code program, all variables used in the G-code must be entered in the G-code variable assignment table, and then the definitions must be transferred to the SRL variable list ([G-code var list > SRL var list] button). This allows the identifiers to be stored with the SRL program. The definitions in the G-code configuration file can be saved to disk using the Configuration general menu.
With an existing SRL program, all variables used in the SRL program must be assigned identifiers and then the SRL variables must be transferred to the G-code variable list ([SRL var list > G-code var list] button). This allows the definitions of the variables used in the G-code to be stored in the G-code configuration file and used during an import.
If different variable definitions are required for several G-code programs, this can be specified in different G-code configurations. These configurations can be saved and reused during the next import or export.
INFORMATION
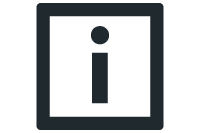
Variables or expressions in the G-code program (as in the SRL program) are not synchronous with the movement. When the program lead-in "P" (program pointer) reaches the expression, it is processed. In the SRL program (e.g. after import) expressions can be synchronized by the WAIT_MOTION_DONE InLastSegment command. Here, the lead-in is stopped until the beginning of the last path segment. Since there is no command in the G-code equivalent to WAIT_MOTION_DONE, the lead-in must be stopped applicatively by programming an IEC call function (M-function). There, the variables Interface_MyRobot.Prg.OUT.lrRemainingDistance and Interface_MyRobot.Prg.OUT.lrRemainingTime can be used by the user code to determine when the lead-in is continued.
Use of pose variables in G-code
Pose variables have 6 dimensions of the "Real" data type. A dimension is accessed via a square bracket after the variable identifier, e.g. Pose_Var_1[ 1..6 ]
. Not every G-code command that expects a numerical value allows the use of a pose variable instead of a real variable. If the pose data type is not allowed for the G-code command used, corresponding information is displayed in the message window of the G-code import dialog. The following example shows the use of pose variables:
Pose_Var_1[3] = Pose_Var_1[3] + 100
Pose_Var_1[3] = Real_Var_1 +100
Pose_Var_1[3] = 100
Vector operations with pose variables (e.g. vector addition Pose_Target = Pose_1 + Pose_2
) are not possible. For example, if vector addition is required, addition must be performed on each dimension of the pose variable:
Pose_Target[1] = Pose_1[1] + Pose_2[1]
Pose_Target[2] = Pose_1[2] + Pose_2[2]
Pose_Target[3] = Pose_1[3] + Pose_2[3]
…
Use of real variables in G-code
The operators +, -, *, / can be used for operations with only real or pose variables. If the dimension is specified for pose variables, both data types can be used as operands:
Real_Target = Real_1 + Real_2
Real_Target = Real_1 / Real_2
Pose_Target[3] = Real_1 + Pose_Target[3]
Real_Target = Pose_1[1] + Pose_2[2]
Implementation of real and pose expressions in the SRL program:
Expressions with numerical operations as well as real and pose variables in the G-code are converted to a CALC_REALVAR block. Signs are allowed for constant numbers, but not for variables. If a constant value should be explicitly displayed as negative in the SRL program, the operator and the sign must be placed in front of the number.
The Real_Target = Real_1 -100
expression is implemented as an SRL command as follows:
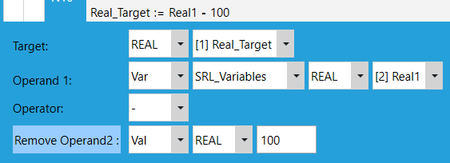
The Real_Target = Real_1 - -100
expression is implemented as an SRL command as follows:
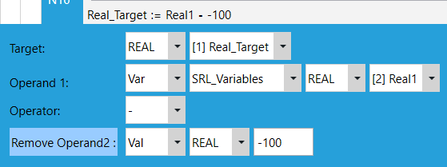
The Real_Target = Real_1 + -100
expression is implemented as an SRL command as follows:
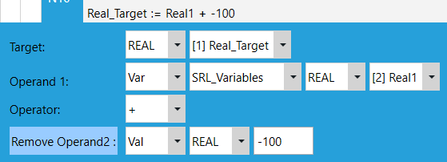
Use of Boolean variables in G-code
BOOL variables can assume the TRUE or FALSE binary state to signal states, for example, or the progress of a G-code program. Boolean expressions can use Boolean variables as well as the TRUE and FALSE constant values. && or AND can be used as operators for "AND", || or OR can be used as operators for "OR" and XOR:
Bool_Target = Bool_1 AND Bool_2
Bool_Target = Bool_1 && Bool_2
The result of comparison operations between real and/or pose variables can be stored in Boolean variables. The == (equal), <> (unequal) and >=, <=, >, < operators are allowed:
Bool_Target = Real_1 == Pose_2[3]
Bool_Target = Real_1 > Pose_2[3]
Bool_Target = Real_1 <> Pose_2[3]
Bool_Target = Real_1 > 100
For Boolean operations, the expression in the G-code is converted to a SET_BOOLVAR block. The specified sign is taken for Boolean operations with numerical operands.
The Bool_Target = Real1 <> -1
expression is implemented as an SRL command as follows:
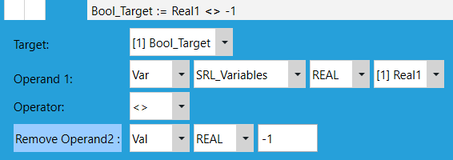
INFORMATION
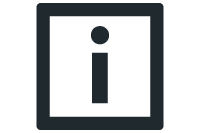
A maximum of two operands are allowed in each operation. If more operands should be contained in an expression, this expression must be divided into several lines as follows:
The phrase Bool_Target = (Pose_1[1] > Pose_2[1]) XOR FALSE
becomes:
Bool_Target = Pose_1[1] > Pose_2[1]
Bool_Target = Bool_Target XOR FALSE
The following graphic illustrates the use of variables in the SRL program: